Welcome to our first tutorial on LearnJavaScripts.com. In this short tutorial, we’ll go through how to set up your developer environment so you can easily follow our other tutorials about web development, particularly JavaScript.
Suppose you already have a developer environment setup. In that case, you can go directly to our next tutorial where we introduce you to JavaScript and give you some basic examples to play around with.
We have structured this tutorial so you can follow it without having any previous experience, that’s why this tutorial is best suited for the absolute rookies. So, let’s get started right away!
Installing Our Development Tools
To get started creating your applications, there are some development tools you first need to install. For this tutorial, we will install VSCode and Nodejs. VSCode is what you will work in and Nodejs is needed to run JavaScript code locally on your computer.
Installing VSCode
For all our tutorials on this website, we’ll use VSCode as the preferred IDE (Integrated Developer Environment).
You can download VSCode directly from https://code.visualstudio.com/download or if you don’t want to install it locally on your computer you can go to https://vscode.dev/ and do all your coding from there. However, it is recommended to install it locally since the web-based version doesn’t support all the features and plugins that the installed version does.
Installing Nodejs
So, the last thing you need to install before we get into the actual coding. You can download Nodejs directly from https://nodejs.org/en/download and we recommend you install the LTS version.
This is the one I installed for this tutorial (I am running 64-bit Windows. You should select the one appropriate to the operating system you use):
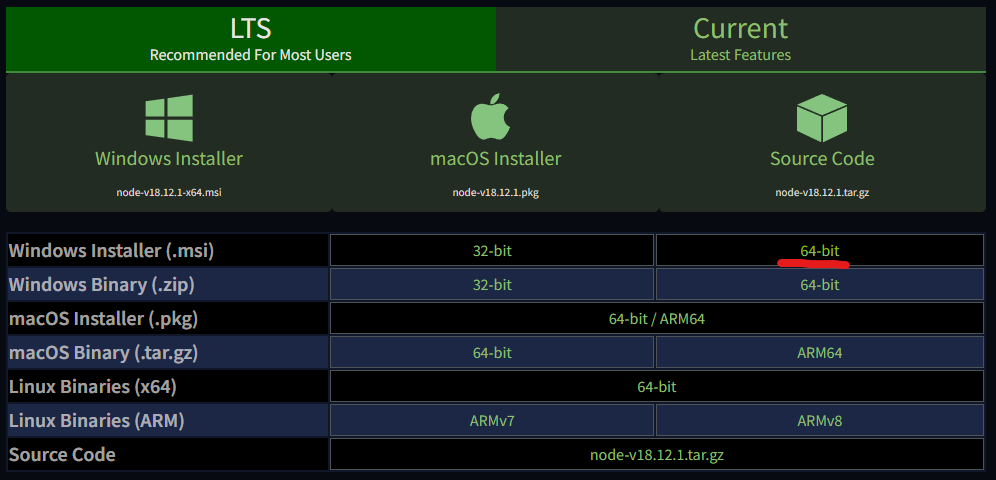
FYI: Node.js comes with npm (Node Package Manager), which allows you to manage JavaScript packages easily. We’ll get more into that in later tutorials 😉
Running Your First JavaScript Code
After you’ve installed VSCode, it’s time to code your first JavaScript program.
Start by creating a new folder and then open up VSCode inside that folder. After you have opened up VSCode inside your newly created folder, click on this option and create a new file, name it main.js
:
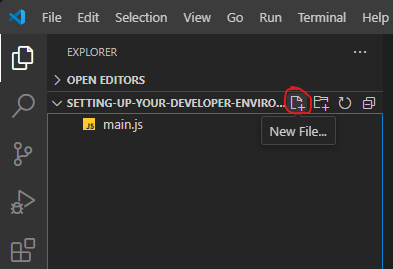
After you’ve created the file, VSCode should automatically open it in the editor view. Add the following line of code to your main.js
file:
console.log("Hello World");
Next, you can click on the Run and Debug option in the left sidebar:
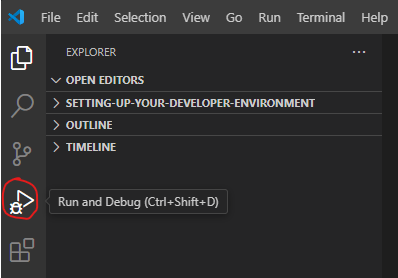
From here, you click on create a launch.json file:
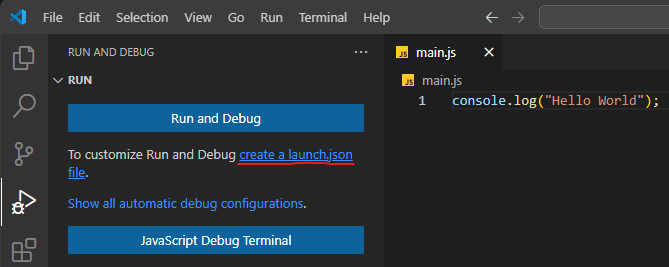
You will now be presented with a couple of options to choose from:
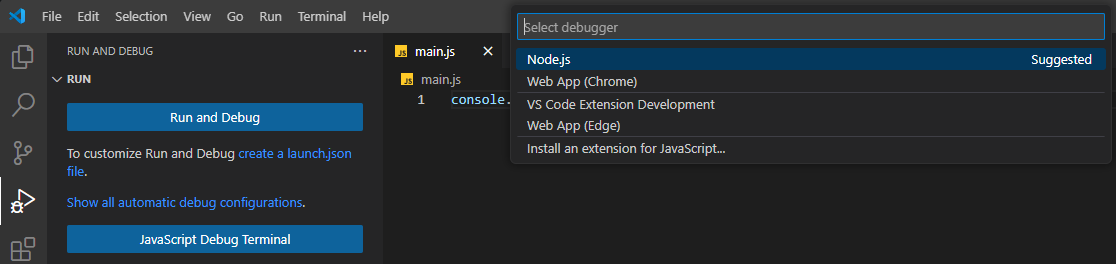
Choose the first option (Node.js). This option is suggested if you want to run your JavaScript code and don’t need a web browser. After you have chosen the Node.js option, VSCode will generate a launch.json
file for you, which will look like this:
{
// Use IntelliSense to learn about possible attributes.
// Hover to view descriptions of existing attributes.
// For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387
"version": "0.2.0",
"configurations": [
{
"type": "node",
"request": "launch",
"name": "Launch Program",
"skipFiles": [
"<node_internals>/**"
],
"program": "${workspaceFolder}\\main.js"
}
]
}
Lastly, click on the Play button inside the Run and Debug menu:
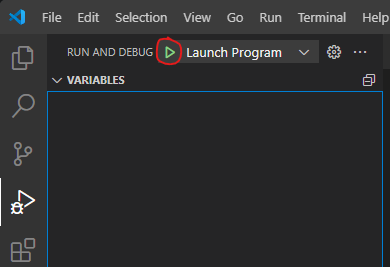
And, here’s the result you get from running your JavaScript code. Cool, right?
C:\Program Files\nodejs\node.exe .\main.js
Hello World
In the next tutorial, we’ll continue introducing you to JavaScript and give you a brief history of the programming language serving most of the web.